today i’m gonna share my personal work which i have done few days ago. so lets start
Parent List = Menu(contains columns: Menu Date, CateringSoups(lookupMulti to insert multiple values) from Soups List, CateringCarving (lookup to insert single item) from Carving list)
Child List = CateringCarving (contains single columns i.e Title)
Child List = CateringSoups(contains single columns i.e Title)
<!-- Start: Site Columns -->
<Field ID="guid" Name="SoupsCM" DisplayName="Soups" Type="LookupMulti" Mult="TRUE" EnforceUniqueValues="FALSE" Required="FALSE" Group="MyCustom Site Columns" />
<Field ID="guid" Name="CarvingCM" DisplayName="Carving" Type="Lookup" Required="FALSE" />
<!--End: Site Columns -->
add these site columns in a content types for me i named it “CateringMenuCT”, then added this content type to list, i always create lookup by modifying in Schema.xml of a list by diving into section, for mine it looks as follow
<Fields>
CateringSoups" ShowField="LinkTitleNoMenu" Type="LookupMulti" Mult="TRUE" EnforceUniqueValues="FALSE" Required="FALSE" Group="custom columns" />
CateringCarving" ShowField="LinkTitleNoMenu" Type="Lookup" Required="TRUE" Group="custom columns" />
</Fields>
see ASPX file
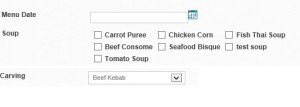
<asp:TextBox ID="dtMenuDate" CssClass="calendar" ClientIDMode="Static" runat="server"></asp:TextBox>
<SharePoint:InputFormCheckBoxList ID="cblSoup" runat="server" RepeatColumns="3" RepeatDirection="Horizontal" />
<asp:DropDownList ID="ddlCarving" runat="server"></asp:DropDownList>
Binding Soups to CheckboxList
SPListItemCollection coll = yourWeb.Lists.TryGetList("CateringSoups").GetItems(query);
currentCheckBoxList.DataSource = coll;
currentCheckBoxList.DataValueField = "ID";
currentCheckBoxList.DataTextField = "Title";
currentCheckBoxList.DataBind();
Binding Carving with DropDownList
SPListItemCollection coll = yourWeb.Lists.TryGetList("CateringCarving").GetItems(query);
ddl.DataSource = coll;
ddl.DataValueField = "ID";
ddl.DataTextField = "Title";
ddl.DataBind();
ddl.Items.Insert(0, new ListItem("", ""));
========================== Saving form data to List =========
SPList list = web.Lists.TryGetList("CateringWeeklyMenu");
SPListItem item = list.AddItem();
item["Soups"] = GetSelectedOptions(cblSoup);
if (!string.IsNullOrEmpty(ddlCarving.SelectedValue))
{
item[Lists.CateringWeeklyMenu.Carving] = new SPFieldLookupValue(ddlCarving.SelectedValue);
}
item.update();
private SPFieldMultiChoiceValue GetSelectedOptions(InputFormCheckBoxList currentCheckBoxList)
{
SPFieldMultiChoiceValue multiValue = new SPFieldMultiChoiceValue();
foreach (ListItem li in currentCheckBoxList.Items)
{
if (li.Selected)
multiValue.Add(Convert.ToString(li.Value) + ";#" + Convert.ToString(li.Text));
}
return multiValue;
}
=========== Loading Values ===========
//Get values from CateringMenu List
SPQuery query = new SPQuery();
query.RowLimit = 1;
SPList list = currentWeb.Lists.TryGetList("CateringWeeklyMenu");SPListItem listItem = coll[0];
string soupsVal = Convert.ToString(listItem["Soups"]);
string CarvingVal = new SPFieldLookupValue(Convert.ToString(listItem["Carving"])).LookupId.ToString();
//Setting back to controls
if (!string.IsNullOrEmpty(CarvingVal) && CarvingVal != "0")
{
ddlCarving.Items.FindByValue(CarvingVal).Selected = true;
}
else
{
ddlCarving.SelectedIndex = 0;
}
SPFieldLookupValueCollection multiValue = new SPFieldLookupValueCollection(SoupsVal);
foreach (SPFieldLookupValue item in multiValue)
{
cblSoup.Items.FindByValue(Convert.ToString(item.LookupId)).Selected = true;
}
happy coding 😉